Java 读取 3124.xlsx Excel 文件 - 处理 16 进制 CardID 和日期格式
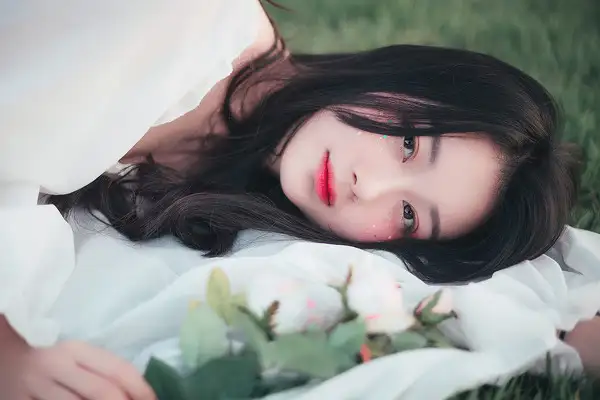
你可以使用 Apache POI 库来读取 Excel 文件。下面是一个示例代码,可以实现你的需求:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class ExcelReader {
private static final String FILE_PATH = 'C:\Users\25346\Desktop\3124.xlsx';
public static void main(String[] args) {
List<CardAttenDence> cardAttenDenceList;
try {
cardAttenDenceList = readExcel(FILE_PATH);
for (CardAttenDence cardAttenDence : cardAttenDenceList) {
System.out.println(cardAttenDence.getCardID());
System.out.println(cardAttenDence.getAttenDatatime());
}
} catch (IOException e) {
e.printStackTrace();
}
}
public static List<CardAttenDence> readExcel(String filePath) throws IOException {
List<CardAttenDence> cardAttenDenceList = new ArrayList<>();
InputStream inputStream = new FileInputStream(filePath);
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheetAt(0); // Assuming the first sheet is the one you want to read
FormulaEvaluator formulaEvaluator = workbook.getCreationHelper().createFormulaEvaluator();
for (Row row : sheet) {
if (row.getRowNum() == 0) {
continue; // Skip the first row (header row)
}
CardAttenDence cardAttenDence = new CardAttenDence();
for (Cell cell : row) {
int columnIndex = cell.getColumnIndex();
CellValue cellValue = formulaEvaluator.evaluate(cell);
switch (columnIndex) {
case 0: // CardID column
if (cellValue.getCellType() == CellType.NUMERIC) {
cardAttenDence.setCardID(Integer.toHexString((int) cellValue.getNumberValue()).toUpperCase());
} else if (cellValue.getCellType() == CellType.STRING) {
cardAttenDence.setCardID(cellValue.getStringValue().toUpperCase());
}
break;
case 1: // AttenDatatime column
if (cellValue.getCellType() == CellType.NUMERIC) {
Date date = cell.getDateCellValue();
SimpleDateFormat dateFormat = new SimpleDateFormat('yyyy-MM-dd HH:mm:ss');
cardAttenDence.setAttenDatatime(dateFormat.format(date));
}
break;
default:
break;
}
}
cardAttenDenceList.add(cardAttenDence);
}
workbook.close();
inputStream.close();
return cardAttenDenceList;
}
}
class CardAttenDence {
private String cardID;
private String attenDatatime;
public String getCardID() {
return cardID;
}
public void setCardID(String cardID) {
this.cardID = cardID;
}
public String getAttenDatatime() {
return attenDatatime;
}
public void setAttenDatatime(String attenDatatime) {
this.attenDatatime = attenDatatime;
}
}
这个示例代码使用 Apache POI 的 XSSFWorkbook 类来读取 xlsx 文件,并使用 FormulaEvaluator 来处理公式计算。在读取每一行和每一个单元格时,根据单元格的类型进行判断和处理。最后,将读取的数据存储在 CardAttenDence 对象中,并添加到 cardAttenDenceList 列表中。
注意:你需要将 Apache POI 的相应依赖添加到项目的类路径中,以便代码能够成功编译和运行。
原文地址: https://gggwd.com/t/topic/pKZ4 著作权归作者所有。请勿转载和采集!