写一个推箱子的Python代码
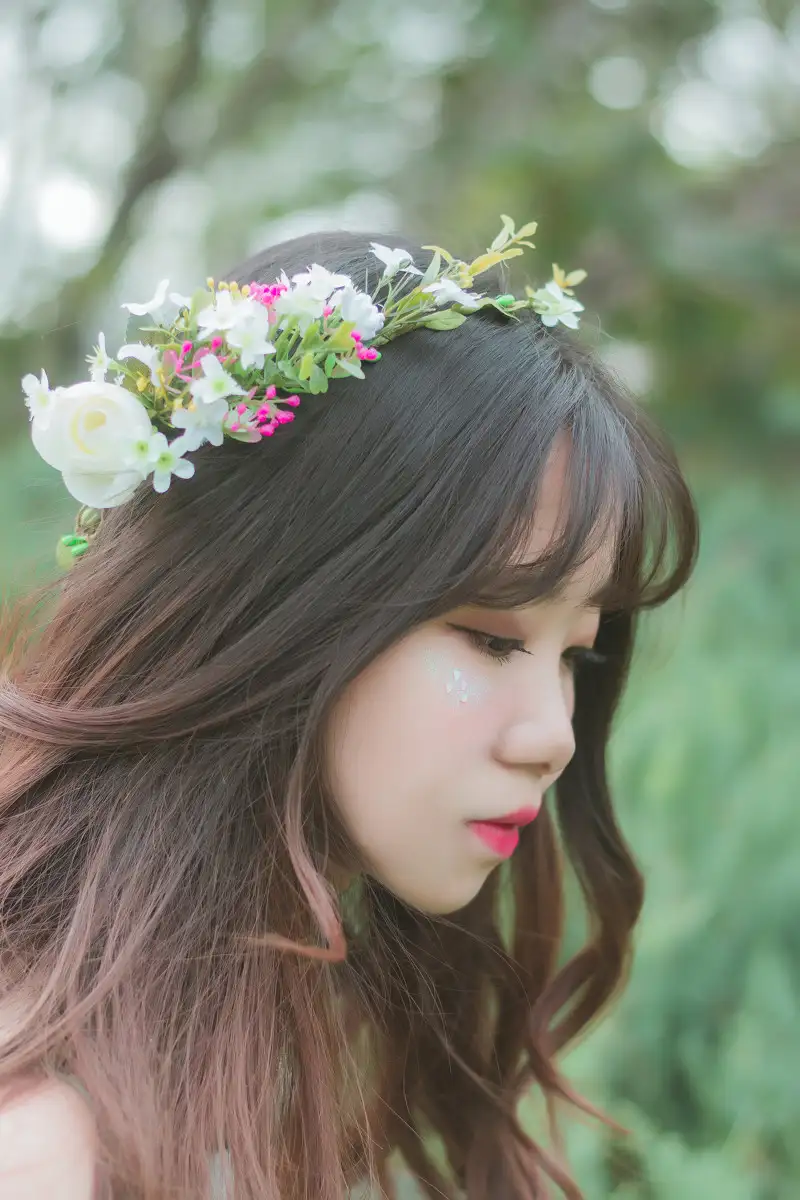
推箱子是一种经典的益智游戏,玩家需要操作角色推动箱子到目标位置。以下是一个简单的推箱子Python代码实现:
# 导入必要的库
import pygame
import sys
# 定义常量
WIDTH = 800
HEIGHT = 600
FPS = 60
BLOCK_SIZE = 50
ROWS = HEIGHT // BLOCK_SIZE
COLS = WIDTH // BLOCK_SIZE
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
BLUE = (0, 0, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
# 定义游戏状态
GAME_OVER = 0
PLAYING = 1
WIN = 2
# 定义游戏地图
MAP = [
"##########",
"# #",
"# #",
"# P #",
"# #",
"# B #",
"# #",
"# #",
"# #",
"##########"
]
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Push Box")
# 加载游戏资源
player_img = pygame.image.load("player.png").convert_alpha()
box_img = pygame.image.load("box.png").convert_alpha()
wall_img = pygame.image.load("wall.png").convert_alpha()
target_img = pygame.image.load("target.png").convert_alpha()
# 定义游戏对象类
class GameObject:
def __init__(self, row, col, img):
self.row = row
self.col = col
self.img = img
def draw(self):
x = self.col * BLOCK_SIZE
y = self.row * BLOCK_SIZE
screen.blit(self.img, (x, y))
# 定义角色类
class Player(GameObject):
def __init__(self, row, col):
super().__init__(row, col, player_img)
def move(self, dx, dy):
new_row = self.row + dy
new_col = self.col + dx
if 0 <= new_row < ROWS and 0 <= new_col < COLS:
if game_map[new_row][new_col] != "#":
self.row = new_row
self.col = new_col
return True
return False
# 定义箱子类
class Box(GameObject):
def __init__(self, row, col):
super().__init__(row, col, box_img)
def move(self, dx, dy):
new_row = self.row + dy
new_col = self.col + dx
if 0 <= new_row < ROWS and 0 <= new_col < COLS:
if game_map[new_row][new_col] != "#" and not is_box(new_row, new_col):
self.row = new_row
self.col = new_col
return True
return False
# 定义游戏地图
game_map = []
player = None
boxes = []
targets = []
for row in range(ROWS):
game_map.append([])
for col in range(COLS):
char = MAP[row][col]
if char == "#":
game_map[row].append("#")
wall = GameObject(row, col, wall_img)
elif char == "P":
game_map[row].append(".")
player = Player(row, col)
elif char == "B":
game_map[row].append(".")
box = Box(row, col)
boxes.append(box)
elif char == " ":
game_map[row].append(".")
elif char == "T":
game_map[row].append(".")
target = GameObject(row, col, target_img)
targets.append(target)
# 判断一个位置是否有箱子
def is_box(row, col):
for box in boxes:
if box.row == row and box.col == col:
return True
return False
# 判断游戏是否胜利
def is_win():
for target in targets:
is_covered = False
for box in boxes:
if box.row == target.row and box.col == target.col:
is_covered = True
break
if not is_covered:
return False
return True
# 游戏循环
state = PLAYING
clock = pygame.time.Clock()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
if player.move(0, -1):
for box in boxes:
if player.row == box.row and player.col == box.col:
box.move(0, -1)
break
elif event.key == pygame.K_DOWN:
if player.move(0, 1):
for box in boxes:
if player.row == box.row and player.col == box.col:
box.move(0, 1)
break
elif event.key == pygame.K_LEFT:
if player.move(-1, 0):
for box in boxes:
if player.row == box.row and player.col == box.col:
box.move(-1, 0)
break
elif event.key == pygame.K_RIGHT:
if player.move(1, 0):
for box in boxes:
if player.row == box.row and player.col == box.col:
box.move(1, 0)
break
# 更新游戏状态
if is_win():
state = WIN
elif state != GAME_OVER and not any([box.row == player.row and box.col == player.col for box in boxes]):
state = GAME_OVER
# 绘制游戏界面
screen.fill(WHITE)
for row in range(ROWS):
for col in range(COLS):
if game_map[row][col] == "#":
wall = GameObject(row, col, wall_img)
wall.draw()
elif game_map[row][col] == ".":
target = GameObject(row, col, target_img)
target.draw()
for box in boxes:
box.draw()
player.draw()
# 绘制游戏状态
if state == GAME_OVER:
font = pygame.font.SysFont(None, 64)
text = font.render("Game Over", True, RED)
screen.blit(text, (WIDTH//2 - text.get_width()//2, HEIGHT//2 - text.get_height()//2))
elif state == WIN:
font = pygame.font.SysFont(None, 64)
text = font.render("You Win!", True, GREEN)
screen.blit(text, (WIDTH//2 - text.get_width()//2, HEIGHT//2 - text.get_height()//2))
# 更新屏幕
pygame.display.update()
clock.tick(FPS)
这个代码实现了一个简单的推箱子游戏,玩家可以使用方向键控制角色移动,并推动箱子到目标位置。游戏界面使用了pygame库来实现,游戏地图使用了一个字符串列表来表示。这个代码仅仅是一个基础实现,可以添加更多的关卡、难度等元素来丰富游戏内容。
原文地址: https://gggwd.com/t/topic/frB 著作权归作者所有。请勿转载和采集!